A HashMap is a part of Java’s collection wherein an index of a type can access store items in key/value pairs. The keys are the unique identifiers used for associating each value on a map. HashMap in Java can access the data much faster and easier.
Every key should be mapped to one value exactly, and it is used to retrieve the corresponding value from a map. If the duplicate key is inserted, the element of the corresponding key is replaced. HashMap in the Java class is found in the java.util package and it provides the implementation of the map interface of Java.
Here are the topics this article will explore regarding HashMap in Java:
- How do HashMaps differ from Hashtable?
- Hierarchy of HashMap
- The Specialty of Constructors in HashMap
- Operations by HashMap in Java
- Internal Structure of HashMap
- Performance of HashMap
How Does HashMap Differ From Hashtable?
S. No. |
HASHMAP |
HASHTABLE |
1 |
HashMap in Java is introduced as a new class in JDK 1.2 and it is the advanced version of Hashtable. |
Hashtable is the legacy class. It was introduced before HashMap. |
2 |
Regarding internal implementation, HashMap allows one null key and multiple null values. |
Hashtable is internally implemented in such a way that it does not allow any null key or null values. |
3 |
HashMap is not thread-safe, so it can’t be shared between many threads. Also, synchronization is not implemented. |
Hashtable can be shared with many threads as it is thread-safe and synchronization is implemented. |
4 |
To traverse the values stored in HashMap, it provides an iterator for iteration. |
Hashtable provides an enumerator to traverse the values stored in it, along with the iterator. |
5 |
HashMap is faster as compared to Hashtable since it does not need the absence of synchronization. |
Hashtable is slower as compared to HashMap in Java because of synchronization. It also eliminates the writing of extra code to get the synchronization. |
6 |
HashMap inherits the Abstract map class. |
Hashtable inherits the Dictionary class. |
7 |
HashMap in Java sorts the mappings based on the ascending order of keys and maintains the insertion order because of the implementation of LinkedHashMap and TreeMap. |
Hashtable doesn’t assure any kind of order. |
What Is the Hierarchy of HashMaps?
The HashMap in the Java class enlarges the AbstractMap and implements the Map interface, as shown below.
MAP |
Implements
ABSTRACTMAP |
HASHMAP |
What Are Constructors in HashMaps?
HashMap in Java has four constructors. Mentioned below are the four types of constructors.
(a) Public HashMap():
It is the most commonly used HashMap constructor. It produces an empty HashMap with a default initial capacity of 16 and a load factor of 0.75.
(b) Public HashMap(int initial Capacity):
It is used to specify the initial capacity and 0.75 ratios. It helps to avoid rehashing the number of mappings to hold the HashMap.
c) Public HashMap(int initial Capacity, float load Factor): It produces an empty HashMap with a specified initial capacity and load factor.
(d) Public HashMap(Map<?extends K,? extends V>m): It creates a Map having the same mappings owing to the specified map. It also comes with a load factor of 0.75.
How Are Various Operations Performed on HashMaps?
The functionalities performed by HashMaps include object creation, entries, addition, retrieving values by passing keys, checking whether the HashMap in Java is empty, element removal, and so on.
(a) Adding Elements:
Put() method is used to add an element to the map. But it will not retain the insertion order. For every element, it generates a separate hash internally, and we index the elements based on the hash to make it more efficient.
(b) Changing Elements:
After adding elements, the change in elements can be done again by adding the element with the put() method. The value of the key can be changed as the elements in the map are indexed using the keys. This can be done by simply inserting the modified value for the key, which needs to be changed.
(c) Removing Elements:
To remove an element from the map, the remove() method can be used. It takes the key-value and removes the mapping for a key.
(d) Traversal of HashMap:
To traverse over any structure of the collection framework, the iterator interface can be used. As iterators work with one type of data that is being used, the next() method can be used to print the entries of HashMap in Java.
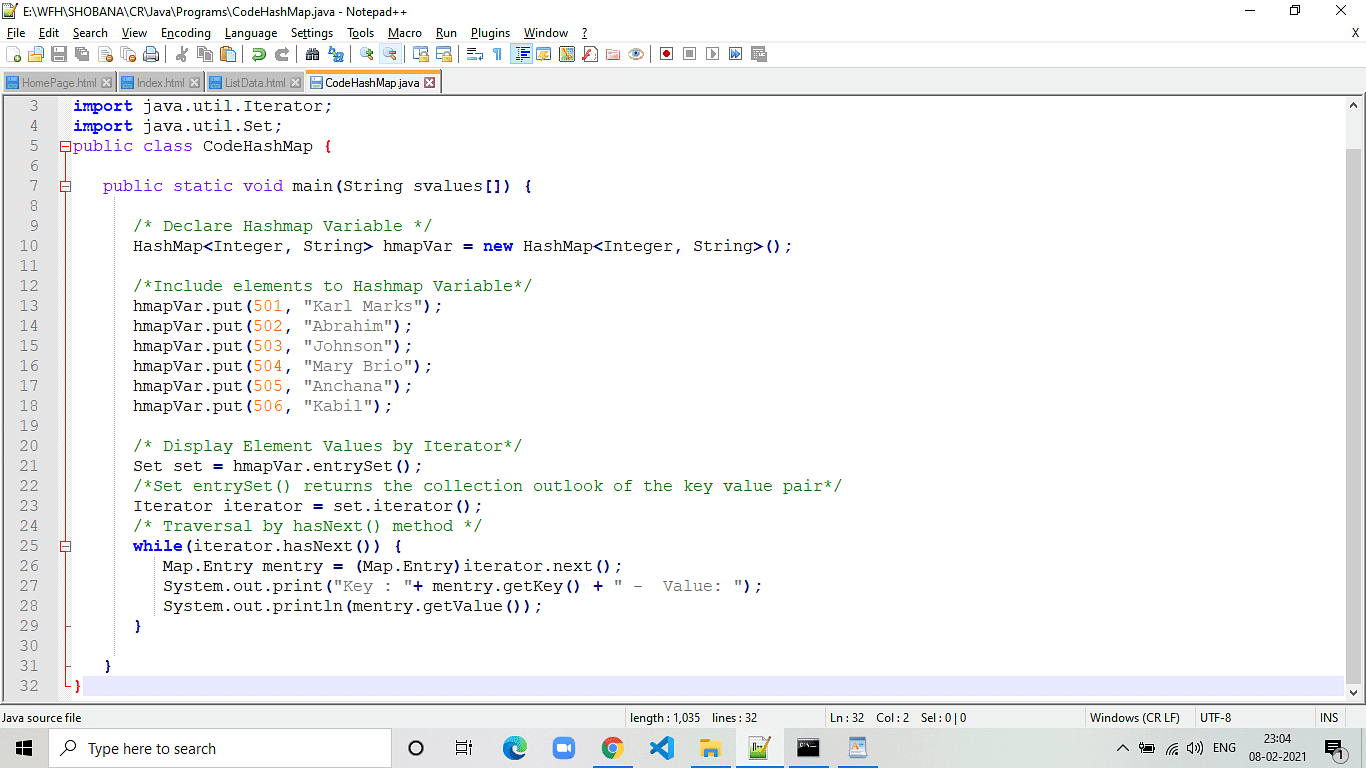
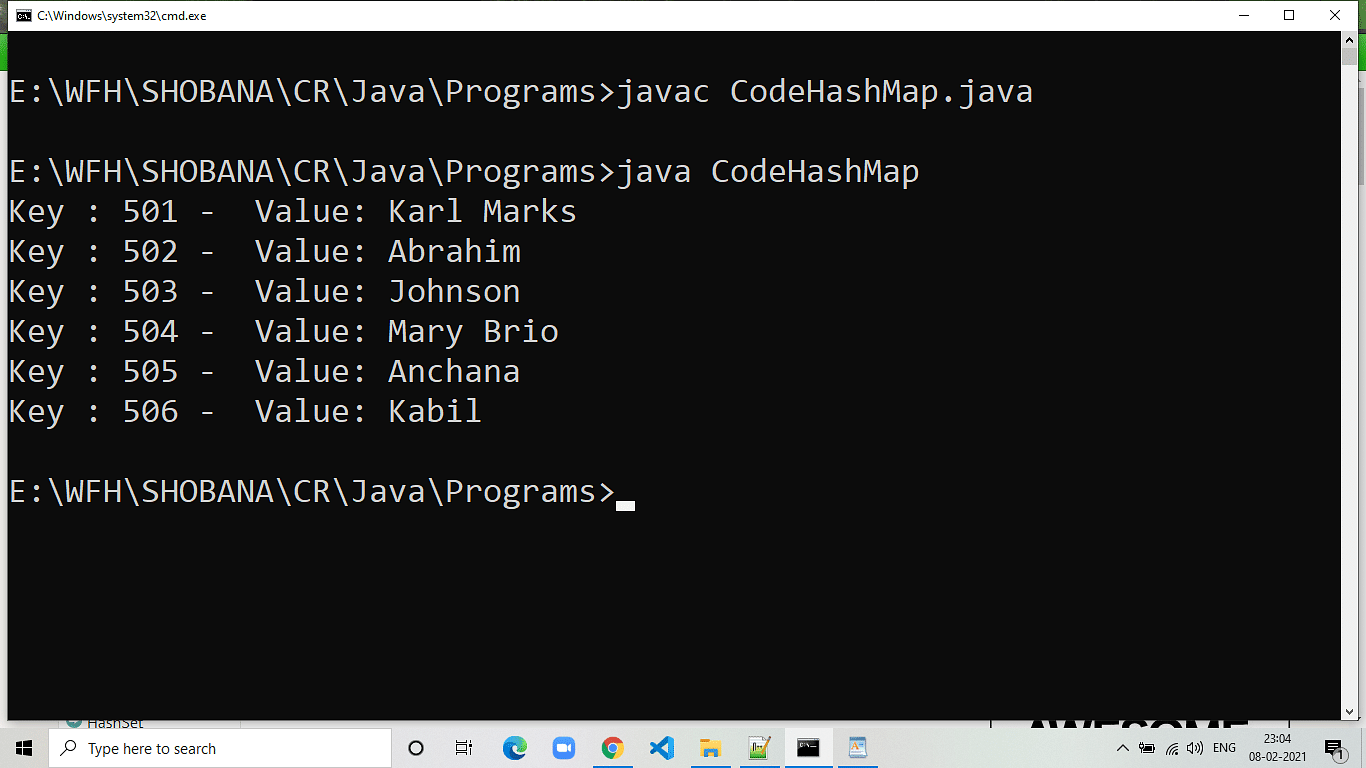
Code:
import java.util.HashMap;
import java.util.Map;
import java.util.Iterator;
import java.util.Set;
public class CodeHashMap {
public static void main(String svalues[]) {
/* Declare Hashmap Variable */
HashMap<Integer, String> hmapVar = new HashMap<Integer, String>();
/*Include elements to Hashmap Variable*/
hmapVar.put(501, "Karl Marks");
hmapVar.put(502, "Abrahim");
hmapVar.put(503, "Johnson");
hmapVar.put(504, "Mary Brio");
hmapVar.put(505, "Anchana");
hmapVar.put(506, "Kabil");
/* Display Element Values by Iterator*/
Set set = hmapVar.entrySet();
/*Set entrySet() returns the collection outlook of the key value pair*/
Iterator iterator = set.iterator();
/* Traversal by hasNext() method */
while(iterator.hasNext()) {
Map.Entry mentry = (Map.Entry)iterator.next();
System.out.print("Key : "+ mentry.getKey() + " - Value: ");
System.out.println(mentry.getValue());
}
}
}
Salient Features and Applications of HashMap
Features:
- HashMap is a part of java.util package
- HashMap in Java extends to an abstract class AbstractMap, which also provides an incomplete implementation of the Map interface
- It implements a cloneable interface that can be serialized
- HashMap in Java doesn’t allow duplicate keys but allows duplicate values. A single key should not contain over one value, but over one key can contain a single value
- It does not assure the order of the map as it’s unsynchronized
- It’s not thread-safe
Applications:
Below are the three main applications of HashMap:
-
Priority Queues
It’s an abstract data type in which each element is assigned a priority. The element that has the highest priority always appears at the front of the queue.
-
Dijkstra’s Algorithm
It helps to find the shortest path from one node to other nodes in a directed weighted graph.
-
Topological sort
Topological order is the ordering of the nodes in the array. It takes a directed graph and returns an array of the nodes, where each node appears before all the nodes it points to.
Internal Structure of HashMap
HashMap in Java internally uses an array to store the elements for each element. It stores four things - hash, key, value, and next. You will find below an image that illustrates how node objects are stored internally in the table array of the HashMap class.
Key1 Value1 Key 2 value 2 null
NULL |
||
NULL |
||
NULL |
||
NULL |
||
Key 3 Value 3 null
Performance of HashMap
In the Java collections framework, the HashMap class implements the interface Map. It has the most constant time performance, and it is one of the high-performance data structures.
When we create a HashMap object, these two mentioned factors influence the performance of HashMap.
1. Initial capacity
Initial capacity is the capacity of HashMap in Java at the time of its creation. The capacity doubles every time it reaches a threshold.
2. Load Factor
It is a measure that decides when to increase the capacity of HashMap. The default value is 0.75f.
Methods in HashMap
There are various methods available in the HashMap class. These methods will help you work with HashMap objects. Let us see the methods in HashMap in detail.
- Void clear(): It removes all the key-value pair mapping from the specified map.
- Int size(): It returns the number of entries available on the specified map.
- Boolean isEmpty(): It checks if the specified map is empty or not. If there are any key and value pairs available, then the function returns false, else true.
- Set entrySet(): It returns the collection outlook of the key-value pair mapping present in the specified map.
- Set keyset() :It returns the collection outlook of keys present in the specified map.
- Value put(Object key,Object value): It inserts a key-value pair mapping into the specified map.
- Void putAll (Map map): It inserts one particular map into the other.
- Boolean containsValue (Object value): It checks the mapping present in the specified map and returns true if there is any value similar to the value being searched, else returns false.
- Boolean containsKey (Object key): It checks the mapping present in the specified map and returns true if there is any key similar to the key being searched for, else returns false.
- Boolean equals (object object): It compares all the values present in the map with the specified object and returns true if it finds an exact match, else returns false.
- Boolean remove (Objectkey): It deletes an entry for the specified key.
- Object clone(): It returns a shallow copy of the HashMap instance.
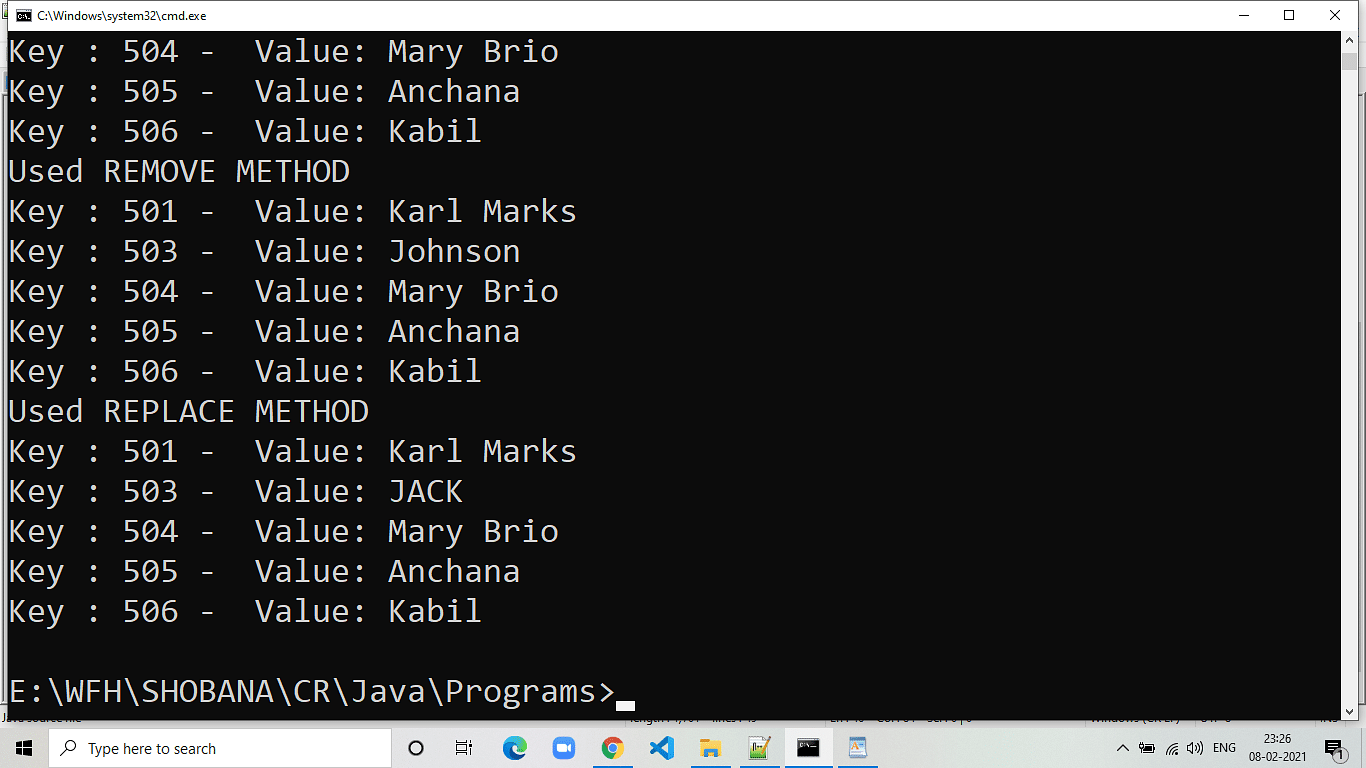
Code:
import java.util.HashMap;
import java.util.Map;
import java.util.Iterator;
import java.util.Set;
public class CodeHashMap {
public static void main(String svalues[]) {
/* Declare Hashmap Variable */
HashMap<Integer, String> hmapVar = new HashMap<Integer, String>();
/*Include elements to Hashmap Variable*/
hmapVar.put(501, "Karl Marks");
hmapVar.put(502, "Abrahim");
hmapVar.put(503, "Johnson");
hmapVar.put(504, "Mary Brio");
hmapVar.put(505, "Anchana");
hmapVar.put(506, "Kabil");
/* Display Element Values by Iterator*/
Set set = hmapVar.entrySet();
/*Set entrySet() returns the collection outlook of the key value pair*/
Iterator iterator = set.iterator();
/* Traversal by hasNext() method */
while(iterator.hasNext()) {
Map.Entry mentry = (Map.Entry)iterator.next();
System.out.print("Key : "+ mentry.getKey() + " - Value: ");
System.out.println(mentry.getValue());
}
/* Remove values based on key*/
hmapVar.remove(502);
System.out.println("Used REMOVE METHOD");
Iterator iterator2 = set.iterator();
/* Traversal by hasNext() method */
while(iterator2.hasNext()) {
Map.Entry mentry2 = (Map.Entry)iterator2.next();
System.out.print("Key : "+ mentry2.getKey() + " - Value: ");
System.out.println(mentry2.getValue());
}
/* Replace values*/
hmapVar.replace(503,"Johnson","JACK");
System.out.println("Used REPLACE METHOD");
for(Map.Entry mtry:hmapVar.entrySet())
{
System.out.println("Key : "+ mtry.getKey() + " - Value: "+mtry.getValue());
}
}
Get a firm foundation in Java, the most commonly used programming language in software development with the Java Certification Training Course.
Conclusion:
HashMap in Java is one of the most powerful data structures which speeds up the process of accessing data. In the Java collection framework, the most used class along with ArrayList is HashMap. It stores key-value pairs and gets the value by using its unique key. HashMap will not allow duplicate keys. When compared to Hashtable, HashMap is faster. The initial capacity and load factor are the two factors that influence the performance of HashMap. You also can work with LinkedHashMap, ConcurrentHashMap, and Treemap along with HashMap.
To sum up, this article covers what a HashMap is, how to use HashMap to its full potential.
For gaining more expertise in Java HashMap, Simplilearn’s java certification program can assist you in broadening your horizon. The course consists of 60-hours of applied learning and has 35 certified coding-related exercises, which will provide you with a first-hand learning experience.
Have any questions for us? Leave them as comments below, and our experts will answer them for you right away!