What Are Packages in Java?
A set of classes and interfaces grouped together are known as Packages in JAVA. The name itself defines that pack (group) of related types such as classes, sub-packages, enumeration, annotations, and interfaces that provide name-space management. Every class is a part of a certain package. When you need to use an existing class, you need to add the package within the Java program.
Why Are They Used For?
The benefits of using Packages in Java are as follows:
- The packages organize the group of classes into a single API unit
- It will control the naming conflicts
- The access protection will be easier. Protected and default are the access level control to the package
- Easy to locate the related classes
- Reuse the existing classes in packages
You can categorize packages into:
- Built-in Packages
- User-defined Packages
The built-in packages are from the Java API. The JAVA API is the library of pre-defined classes available in the Java Development Environment. Few built-in packages are below:
- Java.lang–Bundles the fundamental classes
- Java.io - Bundle of input and output function classes
- Java.awt–Bundle of abstract window toolkit classes
- Java.swing–Bundle of windows application GUI toolkit classes
- Java.net–Bundle of network infrastructure classes
- Java.util–Bundle of collection framework classes
- Java.applet–Bundle of creating applet classes
- Java.sql -Bundle of related data processing classes
The built-in packages are again categorized into extension packages. These extension packages start with javax. This is for all the Java languages, which have lightweight component classes.
- Javax.swing
- Javax.servlet
- Javax.sql
Note: The default package imported with no declaration is java.lang package.
User-defined packages are bundles of groups of classes or interfaces created by the programmer for their purpose.
Packages in Java Working Mechanism
Package_name.sub_package_name.class_name E.g. Built-in packages java.awt.event User-defined packages University.Department.Staff |
In the above example, Java is the package name, awt is the subpackage name and event is the class name. University is the user-defined package name, Department is the subpackage name, and Staff is the class name. Consider a folder inside a file directory. The directory Java is accessible through classpath, to make sure that the classes are easy to locate.
Add Built-in Packages in Java Program
Example 1
Code 1:
import java.util.*; //get all classes from subpackage loads Date class
import java.util.Scanner; //get one specific class Scanner
public class PackageImportExample
{
public static void main(String args[])throws Exception
{
// Instantiate a scanner object
Scanner scanObj = new Scanner(System.in);
System.out.print("Kindly Enter Your UserName :");
String sName = scanObj.nextLine();
System.out.println("UserName is: " + sName);
scanObj.close();
// Instantiate a Date class from Util package
Date currdate = new Date();
System.out.println(currdate.toString());
//Without importing java.net package use complete qualified name to access the class InetAddress
java.net.InetAddress ipAddress=java.net.InetAddress.getLocalHost();
System.out.println("My IP Address :"+ipAddress.getHostAddress());
}
}
To get the use of a package or a class from a library, you need the help of the keyword “import”.
In the above code, there are three ways to import the package.
Import One Specific Class From a Package
- import java.util.scanner
In the above program, you have included only one scanner class from util subpackage. It will get the user value and display.
Import One Whole Package
- import java.util.*
In the above program, the * denotes all the classes from the util package. Along with other classes, it loads the date class as well. The date class displays the current date and time.
Use Complete Qualified Name
java.net.InetAddress ipAddress=java.net.InetAddress.getLocalHost();
The InetAddress class is available in the java.net package, without using the import keyword. Directly call the InetAddress class with the complete package name java.net.InetAddress. This will get the IP address.
Create Your Own Packages in Java
To create a package, you should be aware of the Java file system directory. This is similar to the files and folders organized on your computer. You need the help of the keyword “package” to create your own package. The declaration of the package should be the first statement before any import statements in the Java class.
Before creating your own package, you need to keep in mind that all the classes should be public so that you can access them outside the package.
Let us consider our example of a User-defined package - University.Department.Staff.
Now, create your university package.
Example 2
Code 2:
//creates user defined package university
package university;
public class WelcomeMessage
{
//has one method ShowMessage()
public void ShowMessage()
{
System.out.println("Welcome to our University");
}
}
The above command will create a folder name ‘university’ in the current directory.
Note: –d is used to save the class file in the directory and the ‘.’ (dot) denotes the package in the current directory. To avoid name conflicts, please use lower case for package names.
Let us create the department sub-packages in Java.
A package within another package is a sub-package.
Example 3
Code 3:
//creates sub package within university package
package university.department;
public class DepartmentGoal
{
//has one method DepartmentGoalMessage()
public void DepartmentGoalMessage()
{
System.out.println("Department Message Displayed");
}
}
The above command will create a folder name department inside the folder university.
Let us create the staff class within the department subpackage.
Code 4:
//creates class Staff inside department sub package within university package
package university.department;
public class Staff
{
//has two methods for handling staffs
public void AddStaff()
{
System.out.println("Staff Added !");
}
public void RemoveStaff()
{
System.out.println("Staff Removed !");
}
}
Now the user-defined packages in Java and its class are ready to use. Let us see how to include these classes in the Java program.
Add User-Defined Package in Java Program
By using the keyword “import”, you can add the user-defined packages in Java.
Code 5:
import university.department.Staff;
//import user defined package
public class MyOwnPackageExample
{
public static void main(String args[])
{
Staff mystaff = new Staff();
mystaff.AddStaff();
mystaff.RemoveStaff();
}
}
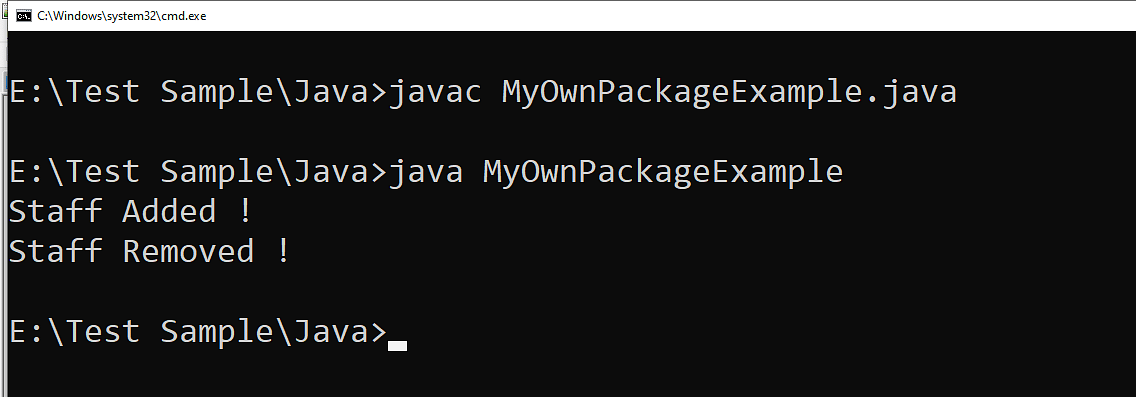
Code 6:
import university.department.Staff;
//import user defined package
import university.department.DepartmentGoal;
public class MyOwnPackageExample
{
public static void main(String args[])
{
//DepartmentGoal class object created by import that class;
DepartmentGoal dgoal =new DepartmentGoal();
dgoal.DepartmentGoalMessage();
Staff mystaff = new Staff();
mystaff.AddStaff();
mystaff.RemoveStaff();
}
}
Note: The import statements should appear after the package statement in package creating files.
Get a firm foundation in Java, the most commonly used programming language in software development with the Java Certification Training Course.
Conclusion:
A Java package is a set of classes, interfaces, and sub-packages that are similar. In Java, it divides packages into two types: built-in packages and user-defined packages.
Built-in Packages (packages from the Java API) and User-defined Packages are the two types of packages (create your own packages). Packages help you prevent name conflicts and write more maintainable code.
For gaining more expertise in Packages in Java, Simplilearn’s Java Certification Training program can assist you in broadening your horizon. The course comprises 250+ hours of applied learning, 20 lesson-end, and 6 phase-end projects, and has 4 industry-aligned capstone projects, which will provide you with a first-hand learning experience.
This Full Stack Java Developer course will teach you the fundamentals of front-end, middleware, and back-end Java web development. You'll learn how to develop applications, test and deploy code, and use MongoDB to store data, among other things.
Have any questions for us? Leave them in the comments section of this article, and our experts will get back to you on them, as soon as possible!